Introduction: In this blog post, we will walk you through the process of creating a traffic light simulator using an Arduino board. This fun project allows you to understand the basics of controlling LEDs and simulate the sequential operation of a traffic light. We will provide a step-by-step guide along with a circuit diagram to help you easily build the project.
Requirements: To complete this project, you will need the following components:
Arduino board (e.g., Arduino Uno)
Breadboard
Three LEDs (Green, Yellow, and Red)
Jumper wires
Circuit Diagram:
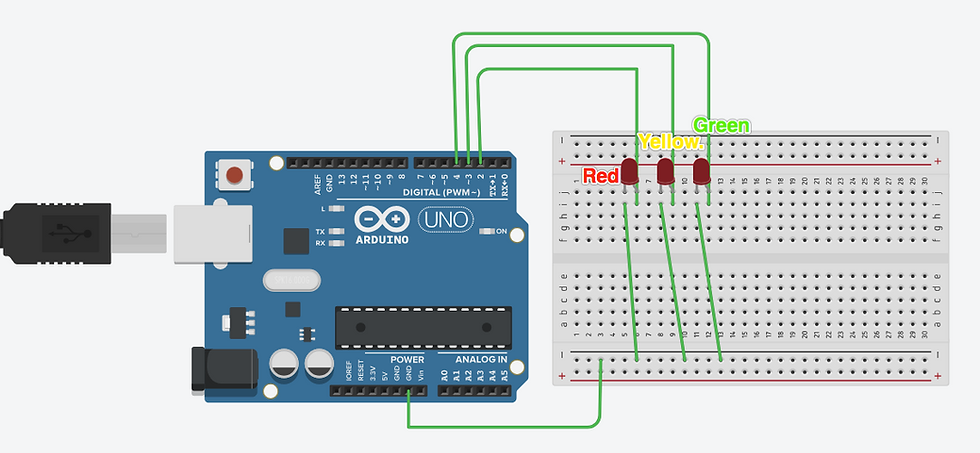
Code:
int greenPin = 2; // Green LED pin
int yellowPin = 3; // Yellow LED pin
int redPin = 4; // Red LED pin
void setup() {
pinMode(greenPin, OUTPUT); // Set green pin as output
pinMode(yellowPin, OUTPUT); // Set yellow pin as output
pinMode(redPin, OUTPUT); // Set red pin as output
}
void loop() {
// Green light
digitalWrite(greenPin, HIGH);
digitalWrite(yellowPin, LOW);
digitalWrite(redPin, LOW);
delay(2500); // Green light duration of 2.5 seconds
// Yellow light
digitalWrite(greenPin, LOW);
digitalWrite(yellowPin, HIGH);
digitalWrite(redPin, LOW);
delay(2000); // Yellow light duration of 2 seconds
// Red light
digitalWrite(greenPin, LOW);
digitalWrite(yellowPin, LOW);
digitalWrite(redPin, HIGH);
delay(2500); // Red light duration of 2.5 seconds
// Yellow light
digitalWrite(greenPin, LOW);
digitalWrite(yellowPin, HIGH);
digitalWrite(redPin, LOW);
delay(2000); // Yellow light duration of 2 seconds
}
Instructions: Follow these instructions to connect the components and build the circuit:
Step 1: Connect the Arduino board to the breadboard.
Place the Arduino board on one side of the breadboard, ensuring that its pins span the center trench.
Step 2: Connect the LEDs to the breadboard.
Insert the green LED's longer leg (anode) into a hole in the breadboard's positive rail.
Repeat this process for the yellow LED (connecting to pin 3) and the red LED (connecting to pin 4) using separate resistors.
Step 3: Connect the LED cathodes to the ground rail.
Connect the cathodes (shorter legs) of all three LEDs to a common row in the breadboard's ground rail using jumper cable.
Step 4: Power the Arduino and upload the code.
Connect the Arduino to your computer using a USB cable.
Open the Arduino IDE and upload the code provided in this blog (ensure the correct pin numbers match your circuit).
Step 5: Test the circuit.
Once the code is uploaded, you should see the traffic light sequence begin: green, yellow, and red in order, with delays between each change.
Conclusion: Congratulations! You have successfully built a traffic light simulator using an Arduino board. This project demonstrates the basics of controlling LEDs and provides a fun way to simulate a traffic light sequence. Feel free to modify the code or add more features to enhance the project further. Enjoy experimenting and exploring the world of Arduino!
Remember to refer to the circuit diagram and instructions provided to ensure correct connections. If you encounter any issues, double-check your connections and code. Happy making!
Comments