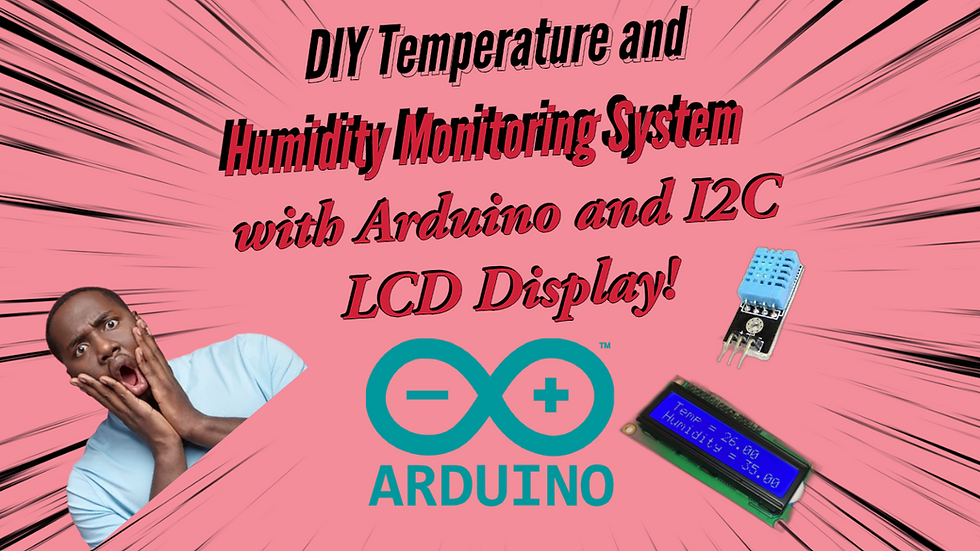
Introduction:
Monitoring temperature and humidity is crucial for various applications, ranging from home automation to environmental control. In this blog post, we will guide you through the process of building a temperature and humidity monitoring system using Arduino and an I2C LCD display. This project will enable you to track and display real-time environmental conditions with ease. So, let's dive in and create your own temperature and humidity monitor using Arduino and I2C communication!
Hardware Components:
Arduino Uno board
DHT11 temperature and humidity sensor
I2C LCD display module
Breadboard and jumper wires
Circuit Diagram: [Insert circuit diagram here]
Step 1: Setting up the Hardware
Connect the DHT11 sensor to the Arduino:
Connect the VCC pin of the DHT11 to 5V on the Arduino.
Connect the GND pin of the DHT11 to GND on the Arduino.
Connect the data pin of the DHT11 to a digital pin on the Arduino (e.g., D2).
Connect the I2C LCD display module to the Arduino:
Connect the SDA pin of the display module to the SDA pin on the Arduino (A4).
Connect the SCL pin of the display module to the SCL pin on the Arduino (A5).
Step 2: Installing the Required Libraries
Open the Arduino IDE.
Go to "Sketch" > "Include Library" > "Manage Libraries".
Search for and install the following libraries:
"DHT Sensor Library" by Adafruit
"LiquidCrystal_I2C" by Frank de Brabander
Step 3: Writing the Arduino Code
#include<DHT.h>
#include <Wire.h>
#include <LiquidCrystal_I2C.h>
#define DHTPIN 2 // Digital pin connected to the DHT11 data pin
#define DHTTYPE DHT11 // DHT11 sensor type
DHT dht(DHTPIN, DHTTYPE);
LiquidCrystal_I2C lcd(0x27, 16, 2); // Set the LCD address and dimensions
void setup() {
lcd.begin(16, 2); // Initialize the LCD display
lcd.backlight(); // Turn on the LCD backlight
dht.begin(); // Initialize the DHT sensor
}
void loop() {
float temperature = dht.readTemperature(); // Read temperature value in Celsius
float humidity = dht.readHumidity(); // Read humidity value
lcd.clear(); // Clear the LCD display
lcd.setCursor(0, 0);
lcd.print("Temp: ");
lcd.print(temperature);
lcd.print("C");
lcd.setCursor(0, 1);
lcd.print("Humidity: ");
lcd.print(humidity);
lcd.print("%");
delay(2000); // Delay for 2 seconds before taking another reading
}
Step 4: Uploading the Code and Testing
Connect your Arduino board to your computer using a USB cable.
Open the Arduino IDE and select the correct board and port under the "Tools" menu.
Copy the code above and paste it into the Arduino IDE.
Click the "Upload" button to upload the code to your Arduino board.
Once the upload is complete, you should see the temperature and humidity readings displayed on the I2C LCD.
Conclusion:
Congratulations! You have successfully built a temperature and humidity monitoring system using Arduino and an I2C LCD display. With this system, you can monitor the environmental conditions in real-time with the convenience of I2C communication. Expand on this project by adding data logging, visualization, or integrating other sensors. Arduino's versatility opens up countless possibilities for your electronics projects.
Remember to explore and experiment further with Arduino's capabilities. With its vast community and extensive library support, you can continue to enhance your projects and learn more about the exciting world of electronics. Enjoy monitoring temperature and humidity with your new Arduino-based system!
Comments